This article was initially printed on .cult by Manusha Chethiyawardhana. .cult is a Berlin-based group platform for builders. We write about all issues career-related, make authentic documentaries and share heaps of different untold developer tales from all over the world.
So that you’re making ready in your subsequent cell improvement interview? Assuming that the corporate is working with the favored cell app improvement framework, React Native, you’re in the precise place.
The interviewer goes to check your React Native information, so let’s be sure you’re ready for any questions coming your method. On this article, I’ll undergo a number of the commonest React Native interview questions and supply the solutions so you possibly can confidently reveal your information of the framework.
1. Why use React Native over different frameworks?
React Native is a cross-platform framework. The usage of a single codebase for 2 platforms (iOS and Android) saves money and time, which is probably the obvious motive to go along with React Native. Nevertheless, it’s not React Native’s solely specialty.
You’ll be able to reveal your in-depth information of React Native by explaining the next causes.
Native Expertise
The Native expertise provision is one of the best characteristic of React Native, versus the cell framework Ionic. As a result of Ionic is constructed on prime of the net browser, utility code can not simply entry native functionalities. Ionic makes use of HTML/CSS, permitting you to name native apps; nonetheless, the smoothness of fundamental capabilities such because the keyboard, maps, navigation, digicam, and so forth can’t be assured.
React Native, however, interacts straight with native UI widgets slightly than utilizing Net View Element or making calls to pre-existing native apps through HTML code or creating replicas of native apps.
Flexibility
React Native doesn’t bind you to its code alone. React Native lets you generate platform-specific code in Xcode if you wish to code natively in Swift. You need to use React Native to compile a local iOS app after which program options straight in iOS utilizing Swift or Goal-C to implement options that aren’t obtainable in React Native or are simpler to implement inside iOS.
React Native can be appropriate with third-party plugins, making it much more versatile. You’ll be able to speed up the event course of even additional by utilizing open-source libraries of pre-built parts. As a result of cross-bridge linking is just not required and a lot of the code is used throughout run-time, it additionally doesn’t devour a lot reminiscence house.
Carry out reside updates with out App Retailer approval
Ready for App Retailer approvals for updates can take a very long time. React Native lets you remotely replace your utility codebase through CodePush from Microsoft App Heart, slightly than going by the whole strategy of submitting the up to date model of your app to the App Retailer after which ready for it to be accredited, as you’d with frameworks like Flutter.
2. What are the Fundamental Elements utilized in a React Native app?
Most React Native apps will use no less than one of many following fundamental parts, which each React Native developer will come throughout.
View — Essentially the most fundamental element for making a React Native UI. VIew helps format with flexbox, type, some contact dealing with, and accessibility controls.
Textual content — A React element for displaying textual content that additionally helps nesting, styling, and contact dealing with.
Picture — A React element for displaying varied kinds of photographs, together with digicam roll photographs.
ScrollView — A scrolling container that may maintain a number of parts and views.
TextInput — The fundamental element for getting into textual content into the app utilizing a keyboard.
StyleSheet — StyleSheet is an abstraction much like CSS StyleSheets that lets you outline types for varied containers.
3. What’s the distinction between ScrollView and FlatList?
ScrollView and FlatList are each used to show an inventory of parts in a React Native app. Nevertheless, ScrollView has a efficiency drawback.
ScrollView will load the entire gadgets as quickly because the element is loaded. Consequently, all information is saved in RAM, and the extra information there’s, the slower the efficiency.
FlatList, however, renders gadgets lazily; by default, it can mount 10 gadgets to the display, and because the consumer scrolls the view, further gadgets will mount.
You need to use ScrollView for a small variety of gadgets and FlatList for a bigger variety of gadgets.
4. Class Elements vs Practical Elements, what to make use of?
Elements in React could be created utilizing both courses or capabilities. Beforehand, solely class parts might have states, however with the introduction of Hooks, now you can use state in operate parts as nicely.
We write fewer traces of code with useful parts, and it’s a lot simpler to learn and take a look at. So, do Class Elements nonetheless must be used?
Sure, there could also be occasions when we have to use Class Elements in trendy React Native apps, equivalent to when dealing with JavaScript errors with Error Boundaries as a result of there is no such thing as a Error Boundaries Hook launched but.
Apart from that, it’s preferable to make use of useful parts when creating your apps.
5. What’s the usage of the useEffect hook?
We are able to carry out uncomfortable side effects in operate parts by utilizing the useEffect Hook. Fetch requests, direct DOM manipulations, and the usage of timer capabilities equivalent to setTimeout() are examples of uncomfortable side effects.
Beforehand, these uncomfortable side effects have been completed by the usage of lifecycle strategies equivalent to componentDidMount(), componentDidUpdate() and componentWillUnmount(). useEffect Hook is a mix of all these strategies. It accepts a callback operate that is known as every time a render happens.
By default, useEffect Hook runs after the primary render in addition to after every replace. The useEffect Hook helps to keep away from redundant code and group associated code.
Take into account the next instance to higher perceive the useEffect Hook for the alternative of the componentDidMount() lifecycle technique.
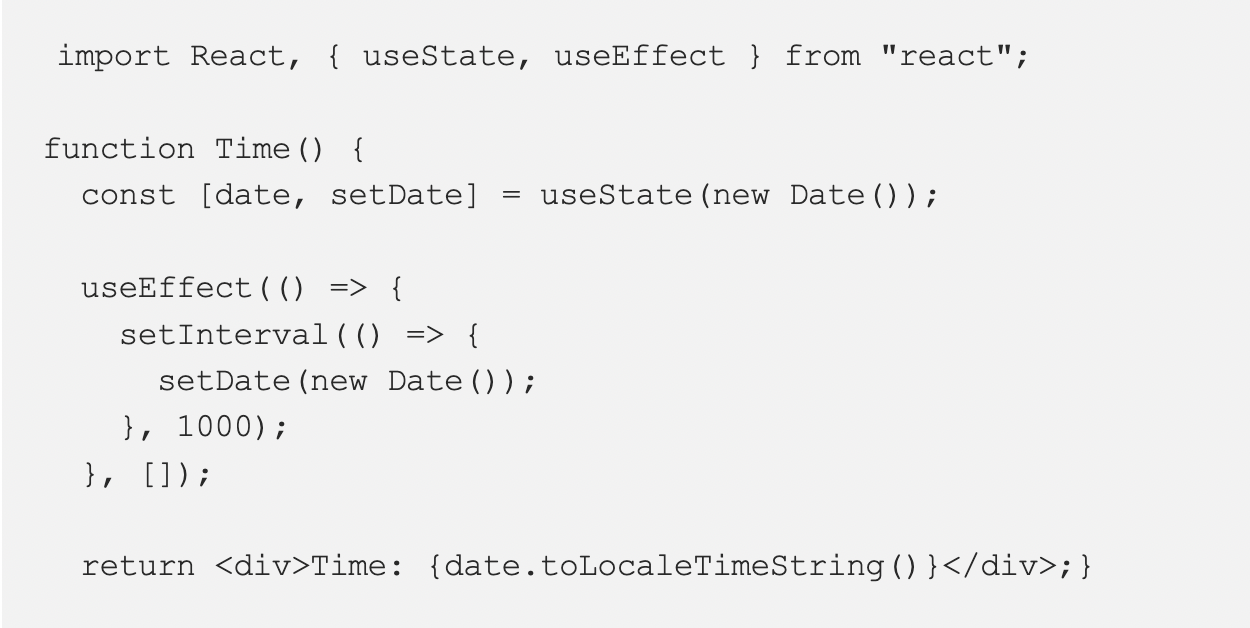
6. What’s Props Drilling and learn how to keep away from it?
Props drilling is the method of passing props from one a part of a tree to a different by passing them by different elements that don’t require the info however solely help in passing it by the tree.
For instance, if we wish to change the theme of the app, we might cross a theme prop by each a part of the element tree, together with elements that don’t require the prop.
Though there’s nothing inherently unsuitable with props drilling, it complicates your utility unnecessarily.
The most effective methods to keep away from props drilling is to make use of the Context API. Context permits us to cross information on to the required parts. We are able to use React Context for props like UI theme, presently authenticated consumer, or language.
7. implement Networking in React Native?
For our networking necessities, React Native consists of the Fetch API and the XMLHttpRequest API. Fetch API is a extra trendy and developer-friendly native JS implementation that makes it simpler to make asynchronous requests and deal with responses.
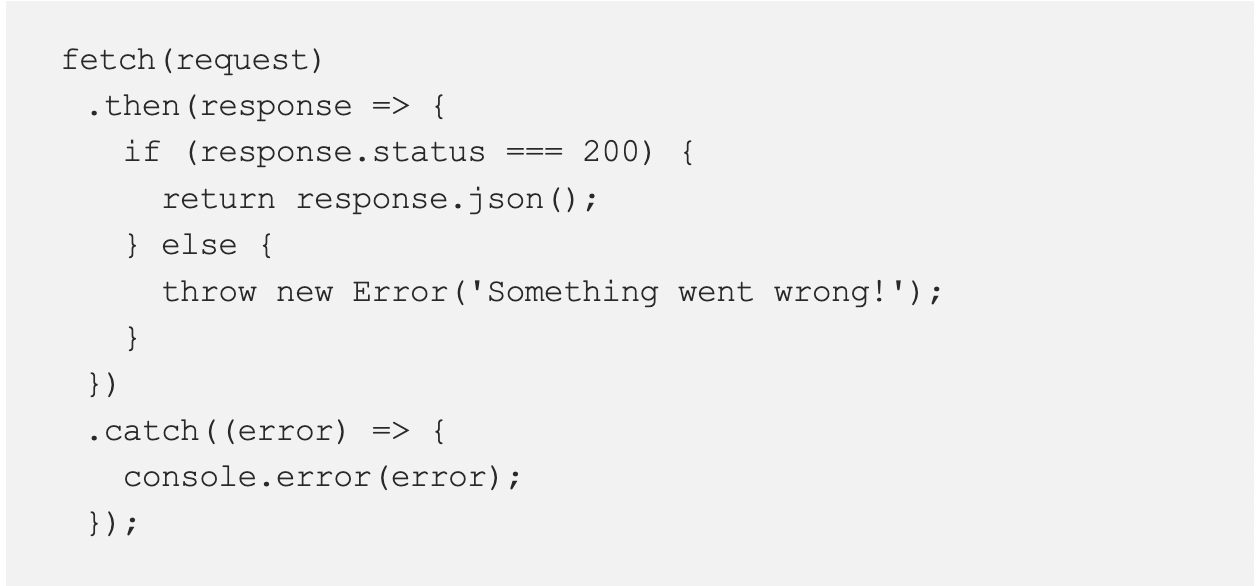
Fetch additionally accepts an optionally available second argument, which permits us to customise the HTTP request. For instance, we are able to use it to specify further headers or to make a POST request.
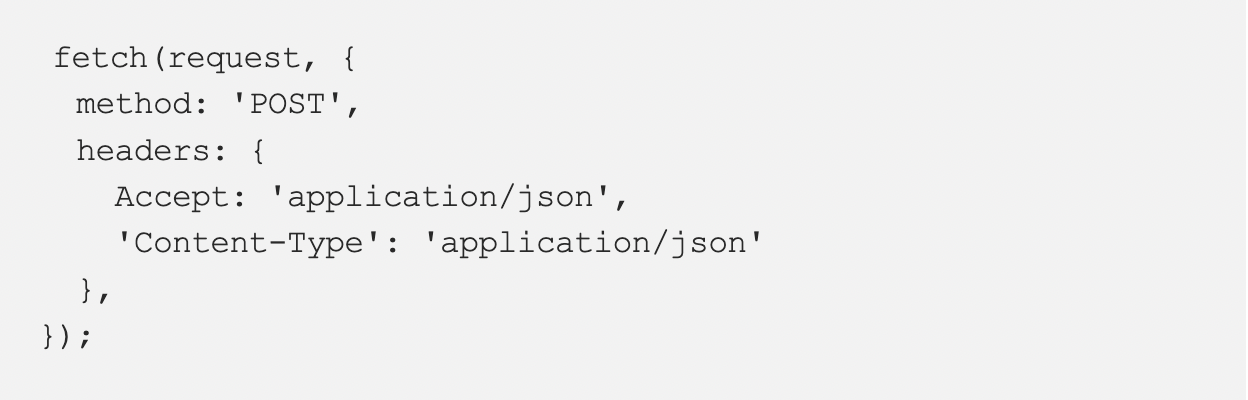
Moreover, we are able to use third-party libraries like axios or frisbee to implement networking in React Native as nicely.
8. What’s Async Storage and when ought to or not it’s used?
Async Storage is a React Native community-maintained module that gives an asynchronous, unencrypted key-value retailer. It’s basically React Native’s model of web-based Native Storage.
Async Storage is beneficial when we have to retailer world app-wide variables, persisting GraphQL or Redux states, or every other non-sensitive information that we wish to preserve even after the consumer closes the app.
As a result of Async Storage can solely retailer string information, object information should be serialized earlier than it may be saved.
Moreover, we should always not retailer delicate information, equivalent to tokens, in Async storage, nor ought to we retailer massive quantities of information.
Github — Async storage react native
9. Why are the animations in React Native so easy?
React Native’s Animated API was designed to be serializable, permitting customers to ship animations to native with out having to undergo the bridge on each body.
As soon as the animation begins, the JS thread could be blocked, and since the code is transformed into native views earlier than rendering, the animations will proceed to run easily.
Earlier than being executed, sure kinds of animation, equivalent to Animated.timing and Animated.spring, could be serialized and despatched throughout the asynchronous bridge.
10. debug React Native purposes?
Many debugging instruments can be found to debug our React Native apps because of numerous React Native contributors.
Chrome DevTools is the only technique to debug your code with out putting in any further apps. React Native by default helps ChromeDev instruments through its distant debugging functionality.
When debugging with React DevTools, you possibly can debug the element hierarchy in addition to choose and edit the element’s present props or state.
In case you’re utilizing Redux in your React Native app, one of the best debugger to make use of is React Native Debugger. It’s a desktop utility that runs on Mac, Home windows, and Linux and combines Redux and React DevTools right into a single app. You need to use React Native Debugger to log or delete Async Storage content material, examine community requests, and detect and diagnose efficiency issues.
Last Ideas
Though there are quite a lot of completely different interview questions you might be requested about React Native, I feel these ten talked about above cowl the commonest subjects. So learn by the solutions once more and ensure ready in your upcoming React Native interview.
In case you discovered these questions useful, let me know and I can put collectively one other listing of extra superior questions! Within the meantime, continue learning and paving the way in which to your dream.
Thanks for studying, and blissful coding!